Top 16 API Security Practices: Safeguarding Your Digital Assets.
- Atharv Karandikar
- Aug 25, 2023
- 13 min read
In today's interconnected digital landscape, Application Programming Interfaces (APIs) are the backbone of seamless communication and data exchange between applications. However, the more interconnected our systems become, the more critical it is to prioritize API security. In this article, we'll explore the top 16 API security practices that are essential for protecting your digital assets from potential vulnerabilities and threats.
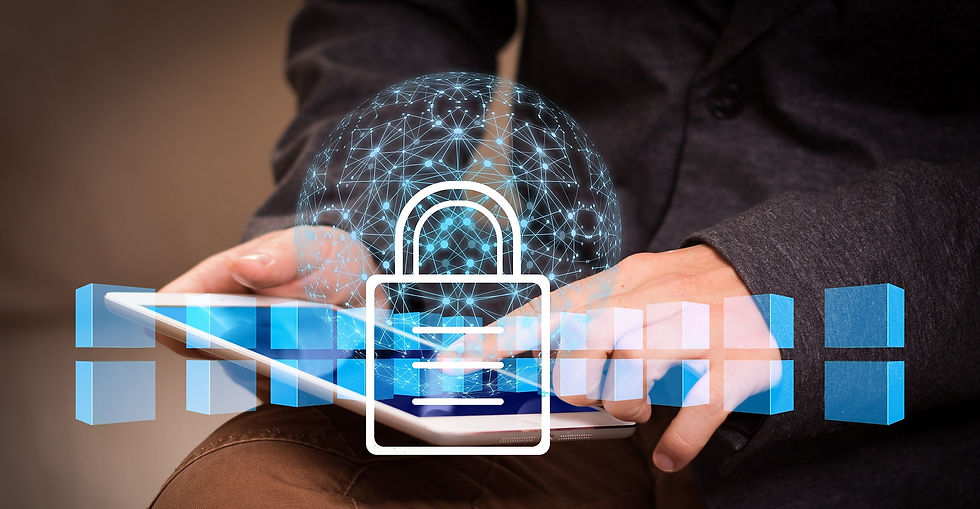
1. Authentication:
Authentication ensures that only legitimate users can access your APIs. Implement strong authentication mechanisms to verify user identities. Authentication is a fundamental aspect of API security. It is the process of verifying the identity of a client or user interacting with an API. The goal is to ensure that the party making the API request is who it claims to be.
There are several methods of authentication used in APIs:
API Keys: These are unique identifiers that are issued to a client. The client must include this key in their API request. While simple and widely used, API keys alone are not considered secure because they can be easily stolen or misused.
Basic Authentication: This involves sending a username and password with each API request. However, this method is not recommended for sensitive data as the credentials are sent in plain text.
Token-Based Authentication: This is a more secure method where the client exchanges credentials for a token, which is then used for authentication during the session. Examples include OAuth and JWT (JSON Web Tokens).
OAuth: This is a token-based method that allows users to authenticate and authorize applications without sharing their credentials. It’s widely used in APIs provided by Google, Facebook, Twitter, and others.
OpenID Connect: This is an identity layer built on top of OAuth 2.0, which allows clients to verify the identity of users based on the authentication performed by an authorization server.
One common approach is API keys:
def authenticate_api(api_key):
valid_keys = ["your_api_key_here"]
if api_key in valid_keys:
return True
return False
2. Authorization:
Authorization defines what actions authenticated users can perform. Utilize role-based access control (RBAC) to manage permissions. Authorization is the second pillar of API security, closely tied to authentication. While authentication is about verifying who is making the request, authorization is about determining what they are allowed to do. General example being Read, Write access to files on a system.
Here are some key points about authorization in API security:
Role-Based Access Control (RBAC): In this model, permissions are associated with roles, and users are assigned to these roles. For example, an ‘admin’ role might have permission to access all endpoints of an API, while a ‘user’ role might only have permission to access a subset.
Attribute-Based Access Control (ABAC): This is a more flexible model where permissions are granted based on attributes of the user, the resource being accessed, and the context of the request. For example, a rule might state that users can only access records that they have created themselves.
Least Privilege Principle: This principle states that a user should be given the minimum levels of access – or permissions – necessary to complete his/her job functions. This principle is applied for security reasons and also for cost-efficiency reasons.
OAuth Scopes: When using OAuth for authorization, scopes can be used to limit an application’s access to a user’s account. A scope is a level of access that an application requests from a user.
API Gateways: These can handle authorization at a central point before requests reach your API. They can offload the responsibility of managing and enforcing access control.
For example:
def check_permission(user_role, required_permission):
role_permissions = {
"user": ["read"],
"admin": ["read", "write", "delete"]
}
return required_permission in role_permissions[user_role]
3. Rate Limiting:
Rate Limiting is a technique for preventing abuse and ensuring fair usage of APIs. It sets a limit on how many requests a client can make to an API in a given amount of time.
Rate limiting can be implemented in following ways:
Per-User Limits: These limits are set based on the user or client making the request. This prevents a single user from monopolizing the API’s resources.
Global Limits: These are overall limits that apply to all requests to the API, regardless of the user. This helps protect the API from being overwhelmed by too many requests at once.
Sliding Window Algorithm: This is a common method for implementing rate limits, where the limit is applied to a continuously moving window of time, rather than resetting at fixed intervals.
429 Status Code: When a client exceeds their rate limit, the API should respond with a 429 ‘Too Many Requests’ status code. The response can also include headers indicating the client’s current usage and when they can make requests again.
API Gateways: These can handle rate limiting at a central point before requests reach your API. They can offload the responsibility of tracking and enforcing rate limits.
Implement rate limiting to prevent abuse and unauthorized access. Throttle requests from a single source using techniques like token bucket:
class TokenBucket:
def __init__(self, capacity, rate):
self.capacity = capacity
self.tokens = capacity
self.last_refill = time.time()
self.rate = rate
def consume(self, tokens):
self.refill()
if tokens <= self.tokens:
self.tokens -= tokens
return True
return False
def refill(self):
now = time.time()
self.tokens = min(
self.capacity, self.tokens + (now - self.last_refill) * self.rate
)
self.last_refill = now
4. Input Validation & Data Sanitization:
Input Validation and Data Sanitization are crucial practices in API security. They help protect your API from malicious input or incorrect data that could lead to security vulnerabilities.
Input Validation: This is the process of checking if the data provided by a user meets certain criteria before it’s processed by the API. For example, ensuring an email address is in the correct format, or a password meets complexity requirements.
Data Sanitization: This involves cleaning or scrubbing input to ensure it’s safe to process. It’s particularly important for preventing injection attacks, where an attacker tries to insert malicious code or commands into their input.
Whitelisting vs Blacklisting: Whitelisting involves defining what is allowed, and rejecting everything else, while blacklisting involves defining what is not allowed, and accepting everything else. Whitelisting is generally considered more secure, as it’s impossible to predict all possible malicious inputs for a blacklist.
Regular Expressions: These can be used to validate input formats. However, they should be used carefully as overly complex regular expressions can lead to Denial of Service (DoS) attacks.
Escaping: This involves making potentially harmful input safe to process, such as converting HTML tags into harmless strings that can’t be executed by a web browser.
Using libraries like OWASP's Java Encoder to sanitize user inputs, Validating and sanitizing input data helps to prevent injection attacks.
import org.owasp.encoder.Encode;
String sanitizedInput = Encode.forHtml(userInput);
5. Encryption:
Encryption is a critical component of API security. It involves converting data into a format that can only be read by someone who possesses the correct decryption key.
Let’s delve into the key aspects of encryption in API security:
Transport Layer Security (TLS): This is a protocol that ensures privacy between communicating applications and their users on the internet. When a server and client communicate, TLS ensures that no third party can eavesdrop or tamper with any message.
End-to-End Encryption: This is a system where only the communicating users can read the messages. In principle, it prevents potential eavesdroppers – including telecom providers, internet providers, and even the provider of the communication service – from being able to access the cryptographic keys needed to decrypt the conversation.
Hashing: While not technically encryption, hashing is related and often used in conjunction with encryption. Hashing creates a unique, fixed-size string of characters from input data of any size. It’s used to ensure data integrity.
Key Management: Securely managing and storing the keys used in encryption is crucial. Keys should be regularly rotated and never hard-coded into your application’s code.
At Rest Encryption: This involves encrypting data when it’s stored, or ‘at rest’, such as in a database. This protects your data in case your storage is compromised.
To Ensure end-to-end encryption of sensitive data. Use HTTPS with TLS to encrypt data in transit. For example, in Node.js:
const https = require('https');
const options = {
hostname: 'api.example.com',
port: 443,
path: '/',
method: 'GET'
};
const req = https.request(options, (res) => {
// Process response
});
req.end();
6. Error Handling:
Error Handling is a crucial aspect of API security. It involves managing and responding to issues that arise during the execution of an API request.
Here are some key points about error handling in API security:
Informative Error Messages: When an error occurs, the API should return a message that accurately describes the problem. However, these messages should not reveal sensitive information that could be exploited by an attacker.
Consistent Error Responses: The structure of error responses should be consistent. This allows clients to easily understand and handle errors.
HTTP Status Codes: APIs should use appropriate HTTP status codes to indicate the type of error. For example, a ‘404 Not Found’ status for a non-existent resource, or a ‘401 Unauthorized’ status for requests lacking proper authentication.
Exception Handling: APIs should have mechanisms in place to catch and handle exceptions, preventing them from causing unwanted side effects or revealing sensitive information.
Logging: All errors should be logged for review and debugging purposes. These logs can help identify patterns, repeated attacks, or areas of the API that are prone to failure.
Rate Limiting on Errors: Implementing rate limiting on endpoints that are producing repeated errors can help protect your API from being overwhelmed and can slow down potential attackers trying to find vulnerabilities.
Implement comprehensive error handling to prevent information leakage, Hence we should only Provide essential information in error responses like example below:
try:
# Code that may raise an exception
except Exception as e:
error_message = "An error occurred"
log_error(e)
return {"error": error_message}, 500
7. Logging and Monitoring:
Logging and monitoring are essential for ensuring the security and performance of any API. Logging is the process of recording events that occur during the execution of an API, such as requests, responses, errors, exceptions, and other relevant information. Monitoring is the process of analyzing the logged data to detect anomalies, trends, patterns, and issues that may affect the API or its consumers.
Logging and monitoring can help API developers and security teams to:
Identify and troubleshoot errors and bugs in the API code or configuration
Detect and respond to malicious attacks or unauthorized access attempts on the API
Measure and optimize the API performance, availability, and reliability
Audit and verify the compliance of the API with security standards and regulations
Gain insights into the usage and behavior of the API consumers and their feedback
API logging and monitoring are important practices for ensuring the security and performance of any API. They help to identify and resolve issues, detect and prevent attacks, measure and optimize performance, audit and verify compliance, and gain insights into the API consumers and their feedback.
8. Security Headers: Security headers are HTTP headers that instruct the browser on how to securely interact with the API. They can help defend against common web attacks, such as cross-site scripting (XSS), clickjacking, content injection, and more. Security headers can also enforce security policies, such as HTTPS-only connections, content security policy (CSP), and strict transport security (HSTS). Some of the best practices for using security headers in APIs are:
Use HTTPS for all API requests and responses, and set the Strict-Transport-Security header to tell the browser to always use HTTPS. For example:
HTTP/1.1 200 OK
Strict-Transport-Security: max-age=31536000;
includeSubDomains
Set the Content-Type header to indicate the format and encoding of the API response, such as application/json or application/xml. For example:
HTTP/1.1 200 OK
Content-Type: application/json; charset=UTF-8
Set the X-Content-Type-Options header to nosniff to stop the browser from guessing the content type and executing malicious scripts. For example:
HTTP/1.1 200 OK
X-Content-Type-Options: nosniff
Set the X-Frame-Options header to deny or sameorigin to prevent the API from being embedded in a frame by another website, which could lead to clickjacking attacks. For example:
HTTP/1.1 200 OK
X-Frame-Options: deny
Set the X-XSS-Protection header to 1; mode=block to activate the browser’s built-in XSS filter and block any malicious scripts. For example:
HTTP/1.1 200 OK
X-XSS-Protection: 1; mode=block
Set the Content-Security-Policy header to define a whitelist of sources and directives for loading scripts, styles, images, fonts, and other resources in the API response. For example:
HTTP/1.1 200 OK
Content-Security-Policy: default-src 'self'; script-src 'self' https://example.com; style-src 'self'
https://example.com; img-src 'self'
https://example.com; font-src 'self'
https://example.com;
Set the Access-Control-Allow-Origin header to specify which origins are allowed to access the API, and use the Access-Control-Allow-Methods, Access-Control-Allow-Headers, and Access-Control-Max-Age headers to control the preflight requests for cross-origin resource sharing (CORS). For example:
HTTP/1.1 200 OK
Access-Control-Allow-Origin: https://example.com
Access-Control-Allow-Methods: GET, POST, PUT, DELETE
Access-Control-Allow-Headers: Content-Type, Authorization
Access-Control-Max-Age: 86400
Set the Cache-Control header to no-store or no-cache to prevent sensitive data from being stored in the browser’s cache. For example:
HTTP/1.1 200 OK
Cache-Control: no-store
By using these security headers we can enhance the security and integrity of APIs and protect digital assets.
9. Token Expiry:
As the name suggests, Token expiry is the process of setting a time limit for the validity of an API token. An API token is a credential that grants access to the API resources and data. Token expiry can help prevent unauthorized access, reduce the risk of token theft, and enforce session management.
Some of the best practices for using token expiry in APIs are:
Use short-lived tokens for high-risk or sensitive operations, and long-lived tokens for low-risk or public operations.
Use refresh tokens to renew expired tokens without requiring the user to re-authenticate.
Use revocation lists to invalidate tokens that are compromised, lost, or no longer needed.
Use HTTPS and TLS to protect the tokens in transit and prevent them from being intercepted and reused by attackers.
Use JWT (JSON Web Token) as the token format for easy verification and validation.
What is JWT?
JWT is a standard format for creating and exchanging tokens. A JWT consists of three parts: a header, a payload, and a signature. The header contains metadata about the token, such as the algorithm used to sign it. The payload contains the claims or information about the user, such as the user ID, roles, permissions, etc. The signature is a cryptographic hash of the header and payload, using a secret key known only to the issuer. The signature ensures that the token has not been tampered with or forged.
Tokens like JWTs should have a limited lifespan. Implement token expiration to minimize their exposure like shown in below example:
import datetime
import jwt
token_payload = {
"user_id": "123456",
"exp": datetime.datetime.utcnow() + datetime.timedelta(hours=1)
}
token = jwt.encode(token_payload, 'secret_key', algorithm='HS256')
10. IP Whitelisting:
IP whitelisting is a technique of securing an API by allowing only specific IP addresses or ranges to access it. IP whitelisting can help prevent unauthorized access, reduce the risk of denial-of-service attacks, and enforce access policies for different clients or users. IP whitelisting can be implemented using various techniques, such as:
Setting up the web server or firewall to filter incoming requests based on their source IP address.
Using a proxy or gateway service that performs IP whitelisting before forwarding requests to the API.
Adding a custom header or parameter to the API requests that contains the client’s IP address and verifying it on the server side.
Using an IP address API, such as ipstack, to get the IP addresses of the API consumers and perform IP whitelisting programmatically.
IP whitelisting is a simple and effective way of securing an API, but it also has some limitations and challenges, such as:
It may not work well with dynamic or shared IP addresses, which can change frequently or belong to multiple clients.
It may require frequent updates and maintenance to keep the whitelist up to date and accurate.
It may not prevent attacks from compromised or malicious clients that have whitelisted IP addresses.
# Import the IPy module
from IPy import IP
# Define a list of whitelisted IP addresses or ranges
myWhiteList = [IP('8.8.x.x'), IP('20x.8x.1xx.0/xx')]
if it is in the whitelist
def is_whitelisted(ip):
for white_net in myWhiteList:
# Check if the IP address is in the whitelisted network
if ip in white_net:
# Return True if yes
return True
# Return False if no
return False
11. Intrusion Detection Systems (IDS):
We all are generally familiar with IDS and their functionalities, Hence, Deploying IDS tools to actively monitor and respond to suspicious activities is a must have security measure. These tools can analyze traffic patterns and identify potential attacks, such as SQL injection attempts or unusual access patterns. An IDS can alert the security team or take action to stop or mitigate the attack. An IDS can help protect APIs from common web threats, such as cross-site scripting (XSS), SQL injection, denial-of-service (DoS), and more. Some of the benefits of using an IDS for APIs are:
It can detect and prevent attacks that exploit unknown or zero-day vulnerabilities in the API code or configuration.
It can provide visibility and insight into the API activity and performance, such as the number, type, and source of requests, responses, and errors.
It can generate logs and reports that can be used for auditing and forensics purposes.
12. Web Application Firewall (WAF):
A WAF provides an additional layer of security by filtering and monitoring incoming API traffic. A WAF can prevent attacks that exploit vulnerabilities in the API code or configuration, such as buffer overflows, parameter tampering, or remote file inclusion. A WAF can also enforce security policies, such as HTTPS-only connections, content security policy (CSP), and strict transport security (HSTS). It can protect APIs from common web attacks, such as cross-site scripting (XSS), SQL injection, denial-of-service (DoS), and more. Therefore, a WAF should be configured and tuned carefully to match the specific needs and characteristics of the API. Some examples of WAF tools for APIs are Cloudflare, AWS WAF, and Imperva.
13. API Versioning:
API versioning is the process of creating and maintaining different versions of an API to accommodate changes in functionality, data model, or design. API versioning can help ensure backward compatibility, avoid breaking changes, and support multiple clients with different needs and expectations. There are different ways to implement API versioning, such as using URI segments, query parameters, headers, or media types.Therefore, API versioning should be planned and implemented carefully to balance between innovation and stability.
14. Data Redaction:
Data redaction is the technique of removing or masking sensitive or personal information from an API response before sending it to the client. Data redaction can help protect the privacy and security of the API consumers and providers, as well as comply with regulations and standards, such as GDPR, HIPAA, or PCI-DSS. Data redaction can be done using various methods, such as encryption, hashing, truncation, substitution, or generalization.
For instance, instead of displaying full credit card numbers, show only the last four digits.
15. Use of Security Standards and Frameworks:
Security standards and frameworks are sets of guidelines and best practices for developing and maintaining secure APIs. They can help improve the quality, consistency, and reliability of the API security design and implementation. They can also help reduce the risk of errors, vulnerabilities, and breaches. Some examples of security standards and frameworks for APIs are OWASP API Security Top 10 and NIST SP 800-204, Some of the benefits of using security standards and frameworks are:
They can provide a common language and reference for API security concepts, principles, and practices among different stakeholders, such as developers, testers, managers, auditors, etc.
They can provide a structured and systematic approach for planning, implementing, testing, reviewing, and improving API security processes and controls.
They can provide a benchmark and a measure for evaluating and comparing the security level and maturity of different APIs.
16. Secure dependencies
Dependencies are any external resources that an application needs in order to function. This includes things like libraries, frameworks, and APIs.
Insecure dependencies:
Insecure dependencies cast a shadow over the foundation of an application's security. These are software components, libraries, or modules that harbor vulnerabilities, which malicious actors can exploit. Such vulnerabilities can range from outdated libraries to unchecked inputs, laying a vulnerable path for cyberattacks like injection attacks, cross-site scripting (XSS), and more. Neglecting to update and monitor these dependencies can expose an application to an array of security breaches.
Secure Dependencies:
API Documentation:
API documentation is the process of creating and maintaining clear and detailed information about an API, such as its purpose, functionality, data model, design, usage, and security. API documentation can help improve the security and quality of an API by:
Providing guidance and instructions for API developers and consumers on how to securely access and use the API resources and data.
Communicating the security policies and requirements of the API, such as authentication, authorization, encryption, rate limiting, etc.
Explaining the security risks and threats that may affect the API or its consumers, such as common web attacks, data breaches, or compliance issues.
Describing the security features and measures that are implemented or recommended to protect the API or its consumers, such as TLS, token expiry, data redaction, etc.
Remember, implementing these practices collectively fortifies your API against potential threats, ensuring the security of both your data and the consumers' data. As the threat landscape evolves, staying updated and proactive with your security measures is essential for maintaining the integrity of your APIs and the trust of your users.
To conclude, What do you call an API that is always secure and never breaks?
A unicorn! 🦄
Because, you know, APIs are hard to secure and maintain, and unicorns are rare and mythical creatures. It’s a pun, hope you get it?
I hope you found this blog/article useful and informative.
Thank you for reading and have a nice day!
Stay Vigilant!
Comments